Adding new logic to already existing components is a common challenge.
Initially, our services start simple, handling just core logic. However, over time new requirements such as logging, caching and more need to be integrated around core logic.
One approach would be to simply modify the existing components including additional logic, but this could lead to:
- Increased Complexity
- Violation of Single Responsibility Principle
- Components larger and harder to maintain
- Testing Difficulties
In today's blog post we will address this issues using Decorator Design Pattern.
Decorator Pattern
Decorator Pattern is a structural design pattern that enables adding behavior to an object without modifying its original code.
Instead of altering the existing class, you wrap it inside another class (a decorator) that provides additional functionality.
This pattern is particularly useful when you need to extend the behavior of components like repositories or services without modifying them directly.
You can also have multiple decorators, that can handle different concerns separately and stack them as needed.
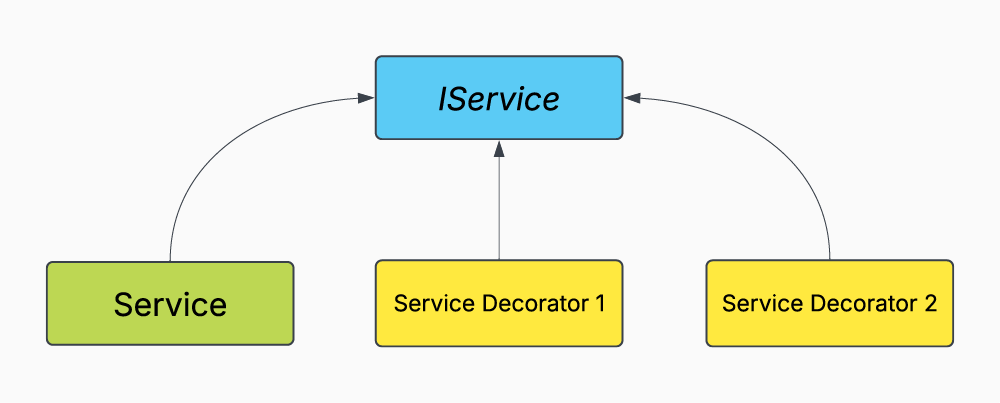
Let's see how this pattern can be implemented in our code.
Implementation
For this example, I’ve created a simple Weather Forecast service class that implements IWeatherService interface and returns a list of random weather forecasts:
public class WeatherService : IWeatherService
{
public IEnumerable<WeatherForecast> GetWeatherForecast()
{
var forecast = Enumerable.Range(1, 5)
.Select(index => new WeatherForecast(
DateOnly.FromDateTime(DateTime.Now.AddDays(index)),
Random.Shared.Next(-20, 55),
_summaries[Random.Shared.Next(_summaries.Length)]));
return forecast;
}
}
This service is registered in dependency injection like this:
builder.Services.AddTransient<IWeatherService, WeatherService>();
Now, to add logging without modifying the original WeatherService, we can create a decorator class that wraps our base service:
public class LoggingWeatherServiceDecorator(
ILogger<LoggingWeatherServiceDecorator> logger,
IWeatherService decoratedService) : IWeatherService
{
public IEnumerable<WeatherForecast> GetWeatherForecast()
{
logger.LogInformation("Executing {MethodName} - Start",
nameof(GetWeatherForecast));
var result = decoratedService.GetWeatherForecast();
logger.LogInformation("Executing {MethodName} - Completed",
nameof(GetWeatherForecast));
return result;
}
}
The decorator class also implements IWeatherService and injects the actual base service through its constructor.
Within its methods, we can introduce additional logic before and after invoking the original service.
The final step is to register the decorator correctly in the dependency injection container.
Manual Configuration
To resolve IWeatherService, we need to modify our existing configuration.
First, we should register WeatherService directly, without using IWeatherService, then we need to register IWeatherService as LoggingWeatherServiceDecorator using service provider to manually create instance.
builder.Services.AddTransient<WeatherService>();
builder.Services.AddTransient<IWeatherService>(sp =>
{
var service = sp.GetRequiredService<WeatherService>();
var logger = sp.GetRequiredService<ILogger<LoggingWeatherServiceDecorator>>();
return new LoggingWeatherServiceDecorator(logger, service);
});
Another approach would be to register IWeather service like this:
builder.Services.AddTransient<IWeatherService>(sp =>
{
var logger = sp.GetRequiredService<ILogger<LoggingWeatherServiceDecorator>>();
return new LoggingWeatherServiceDecorator(logger, new WeatherService());
});
I find the first approach a bit cleaner, however both served me well.
Configuration using Scrutor
Scrutor is an open source library I often use when dealing with assembly scanning and decoration.
This library adds 2 extension methods to IServiceCollection, Scan and Decorate.
Decorate is used to decorate already registered services and here is how it will simplify the configuration:
builder.Services.AddTransient<IWeatherService, WeatherService>();
builder.Services.Decorate<IWeatherService, LoggingWeatherServiceDecorator>();
Conclusion
The Decorator Pattern is a powerful way to extend functionality while keeping code maintainable.
While manual registration works well, using Scrutor simplifies the configuration, making it easier to apply decorators, especially if there are multiple.
If you want to check out examples I created, you can find the source code here:
Source CodeI hope you enjoyed it, subscribe and get a notification when a new blog is up!